Tagged "web programming"
When Code Duplication is not Code Duplication
Duplicating code is a bad thing. Any engineer worth his salt knows that the more you repeat yourself, the more difficult it will be to maintain your code. We've enshrined this in a well-known principle called the DRY principle, where DRY is an acronym standing for Don't Repeat Yourself. So code duplication should be avoided at all costs. Right?
At work I recently came across an interesting case of code duplication that merits more thought, and shows how there is some subtlety needed in application of every coding guideline, even the bedrock ones.
Consider the following CSS, which is a simplified version of a common scenario.
.title {
color: #111111;
}
.text-color-gray-1 {
color: #111111;
}
This looks like code duplication, right? If both classes are applying the same color, then they do the same thing. If the do the same thing, then they should BE the same thing, right?
But CSS and markup in general presents an interesting case. Are these rules really doing the same thing? Are they both responsible for making the text gray? No.
The function of these two rules is different, even though the effect is the same. The first rule styles titles on the website, while the second rule styles any arbitrary div. The first rule is a generalized style, while the second rule is a special case override. The two rules do fundamentally different things.
Imagine a case where we optimized those two classes by removing the title class and just using the latter class. Then the designer changes the title color to dark blue. To change the title color, the developer now has to replace each occurrence of .text-color-gray-1 where it styles a title. So, by optimizing two things with different purposes, the developer has actually made more work.
It's important to recognize in this case that code duplication is not always code duplication. Just because these two CSS classes are applying the same color doesn't mean that they are doing the same thing. In this case, the CSS classes are more like variables than methods. They hold the same value, but that is just a coincidence.
What looks like code duplication is not actually code duplication.
But… what is the correct thing?
There is no right answer here. It's a complex problem. You could solve it in lots of different ways, and there are probably three or four different approaches that are equally valid, in the sense that they result in the same amount of maintenance.
The important thing is not to insist that there is one right way to solve this problem, but to recognize that blithely applying the DRY principle here may not be the path to less maintenance.
Single File Components are Good
I was reading the Vue.js documentation recently, and I came across a very compelling little passage. The passage was explaining the thinking behind single file components. In a single file component, the template, javascript logic, and perhaps even styles, are all collocated in the same file.
Putting all the parts of a component into a single file may seem odd to some programmers. After all, shouldn't we be separating logic from templates? Shouldn't we be separating styles from logic too?
And the answer is that the right thing to do is situation dependent, and this is something that a lot of modern programmers don't understand. A lot of programmers seem to think that there are rules, and you must follow the rules. They don't realize that rules should only be followed when they are helping you.
So the core question is this: is separating a single view into several files really helping your development process?
Here's what the Vue documentation says.
One important thing to note is that separation of concerns is not equal to separation of file types. In modern UI development, we have found that instead of dividing the codebase into three huge layers that interweave with one another, it makes much more sense to divide them into loosely-coupled components and compose them. Inside a component, its template, logic and styles are inherently coupled, and collocating them actually makes the component more cohesive and maintainable.
So the argument from the Vue developers is that keeping all the code related to one view inside one file is actually more maintainable than separating them.
I tentatively agree with this.
Obviously, it's good to separate certain things out into their own files. Foundational CSS styles and core JS helper methods should have their own place. But for all the code that makes a component unique, I think it's easiest for developers if that is in one file.
Here are the problems I face with breaking a view into multiple files.
1. What is each file called? Where is it located?
When you separate a view into multiple files, it becomes difficult to find each file. It's fine to have a standard for naming and locating files, but in any large software project, there are going to be situations where the standard can't be followed for one reason or another. Maybe the view was created early in development. Maybe the view is shared. Or maybe the view was created by a trainee developer and the mis-naming was missed in the code review. Whatever the cause, file names and locations can be stumbling blocks.
At my workplace, we have hundreds of templates named item.js.hamlbars. That's not very helpful, but what's more troublesome is when, for whatever reason, a list item couldn't be named item.js.hamlbars. In that case, I'm left searching for files with whatever code words I can guess.
2. Where is the bug?
When you separate a view into multiple files, you don't know where a bug may be coming from. This is especially troublesome if you are in a situation where conditional logic is being put into the templates. Once you have conditional logic in the templates, then you can't know if the bug is in the javascript or in the template itself. Furthermore, it then takes developer time to figure out where to put the fix.
Single File Components are a Bonus
Putting all the code for a single view into a single file is not only logical, but it also doesn't violate the separation-of-concerns ethos. While the pieces are all in the same file, they each have a distinct section. So this isn't the bad old days of web development, where javascript was strewn across a page in any old place. Rather, collocating all the code files for a single view is a way to take away some common development headaches.
Redux in one sentence
Redux is a very simple tool that is very poorly explained. In this article, I will explain Redux in a single sentence that is sorely missing from the Redux documentation.
Redux is a pattern for modifying state using events.
That's it. It's a dedicated event bus for managing application state. Read on for a more detailed explanation, and for a specific explanation of the vocabulary used in Redux.
1. Redux is a design pattern.
The first thing you need to know is that Redux is not really software per se. True, you can download a Redux "library", but this is just a library of convenience functions and definitions. You could implement a Redux architecture without using Redux software.
In this sense, Redux is not like jQuery, or React, or most of the libraries you get from npm or yarn. When you learn Redux, you aren't learning one specific thing, but really just a group of concepts. In computer science, we call concepts like this a design pattern. A design pattern is a group of concepts that simplify an archetypal task (a task that re-occurs in software development). Redux is a design pattern for managing data in a javascript application.
2. Redux is an extension of the Observer Pattern.
I think this is the only bullet point needed to explain Redux to experienced developers.
The observer pattern is the abstract definition of what programmers refer to as events and event listeners. The observer pattern defines a situation where one object is listening to events triggered by another object.
In javascript, we commonly use the observer pattern when we wire up onClick events. When you set up an onClick event, you are saying that an object should listen to an HTML element, and respond when it is clicked. In this scenario, we are usually managing the visual state of the page using events. For example, a switch is clicked and it must respond by changing color.
Redux is a dedicated event bus for managing internal application state using events.
So redux is a way of triggering pre-defined events to modify state in pre-determined ways. This allows us to ensure that state isn't being modified in ad hoc ways and it allows us to examine the history of application state by looking at the sequence of events that led to that state.
3. Actions are event definitions. Reducers are state modifiers.
The new vocabulary used by Redux makes it seem like it's something new, when it's really just a slight twist on existing technology and patterns.
Action = Event definition. Like events in any language, events in Redux have a name and a payload. Events are not active like classes or methods. They are (usually) passive structures that can be contained or transmitted in json.
Reducer = Event listener. A reducer changes application state in response to an action. It's called a Reducer because it literally works like something you would pass to the reduce function.
Dispatch = Trigger event. The dispatch method can be confusing at first, but it is just a way of triggering the event/action. You should think of it like the methods used to trigger exceptions in most languages. After all, exceptions are just another variation on the Observer pattern. So dispatch is like throw in Java or raise in Ruby.
4. Redux manages local state separately from the backend.
Redux is a pattern for managing only the internal state of the application. It does not dictate anything about how you talk to your API, nor does it dictate the way that your internal state should be connected to the backend state.
It's a common mistake to put the backend code directly into the Redux action or reducer. Although this can seem sensible at times, tightly coupling the local application state with backend interactions is dangerous and often undesirable. For instance, a user may toggle a switch three or four times just to see what it does. Usually you want to update local state immediately when they hit the switch, but you only want to update the backend after they have stopped.
Conclusion
Redux is not complex. It is a dedicated event bus for managing local application state. Actions are events. Reducers modify state. That's it. I hope that helps.
Here are a few other useful links:
https://alligator.io/redux/redux-intro/ https://www.tutorialspoint.com/redux/redux_core_concepts.htm https://redux.js.org/introduction/core-concepts/
How does the internet work?
In this post, I'm going to give you a simple explanation of how the internet works. This is the first in a series of posts that introduce readers to modern web programming.
TLDR: It's just computers talking to each other
When using the internet, one device requests a piece of information that is stored on a computer connected to the internet, and that computer returns it to the requesting device. The modern web is much more complex than that, but at it's simplest level it's just computer A requesting information from computer B.
The requesting device is usually called the client. The computer that serves the request is usually called a server. So if you have to remember one thing about how the web works, just remember this:
Clients request information that is stored on servers. Servers return that information to the clients.
What is a client?
The "client" we're actually talking about is a web browser. The web browser knows how to do several things. Here's some of the things that a web browser can do.
- Build a request for information using a language, or protocol, called HTTP.
- Send that request to servers on the internet by using the operating system to access network resources.
- Parse a response to an HTTP request that sometimes includes a web page.
- Display a web page made of html, css, and javascript.
- Interpret events fired by the operating system that come from devices such as keyboards, touchscreens, and mice.
That's just a bare fraction of what the client does, but it shows that modern web browsers are technical marvels.
What is a server?
The job of the server is slightly different. The server runs a piece of software that listens snd responds to incoming web requests. Such software is called a web server and it includes Apache, Node, and nginx. When it receives a web request, it seeks the resource that corresponds to the requested information to try to fulfill the request.
In the very early days of the web, the resources were just files. So a client might make a request for "/schedule.txt" and the server would see if it had such a file, and return it if it did.
How does the client know where to find the server?
Before the client can make a request to a server, it has to know which server has the information that it is seeking. It does this by using a service called Domain Name System, or just DNS for short.
Before the web browser makes the main web request, it makes an initial request to your internet service provider, or ISP, to ask where to find that information. So let's look at how this might work when you request "google.com".
- The web browser transforms "google.com" into "http://www.google.com". The "http" stands for Hyper Text Transfer Protocol, and that's the format of the request. The "www" is just an old fashioned naming system that stands for "World Wide Web". When someone sets up a "www" subdomain, they're essentially saying that that address should be publicly available on the internet.
- The web browser then sends a request to your DNS to learn the address for "http://www.google.com". That request goes to a DNS server, which responds with an IP address, which is essentially like a telephone number for a computer. So the request for the address for "http://www.google.com" might return something like "123.456.78.910".
- The web browser then sends a request to the indicated IP address, "123.456.78.910". Then the server does whatever it needs to do to fulfill the request, more on this later, and returns the requested information to the client.
- The client renders the page returned from the server.
Okay, so this is getting a little bit more complex, but it still follows the same model. The web browser first sends a web request to the DNS, then it sends the web request for the specific piece of information.
What does the server actually do?
There is a lot that I am glossing over in this explanation, because I'm trying to keep it simple. I will write future posts that go into a little more detail about how the client and server work, and show how code comes into play in those processes.
But we can break the action of the web server into several steps.
- It validates the request to ensure that it's a request that it can fulfill.
- It locates the requested resource. If no resource is requested, then it seeks a default, which is usually "index.html".
- It reads the requested resource, checking to see if it needs any additional information.
- It uses attached data sources such as databases or caches to request information needed to render the resource.
- It builds the final response incorporating the requested resource as well as any data requested from data sources.
But how does it actually put all of this together? It's the job of a web programmer to tell the server how to assemble a web page that fulfills the user's request.
I want to restate that because that's why we study the web. We care about how the web works so that we can contribute to making it more useful.
It's the job of web programmers to write instructions for web servers to fulfill web requests.
Summary
In this post, I gave a concise overview of how the internet works. Here are the main takeaways.
- When using the internet, a client makes a request for a resource that is stored on a server.
- The client knows how to make web requests and render the response using html, css, and javascript.
- The server uses web software to build web pages using by filling in information from connected data sources.
- DNS servers tell web browsers where to find the web address they are seeking.
More
How to set up a computer for web development
In this post, I'm going to suggest a basic set of tools required for web development. These instructions are aimed at people who are using a desktop computer for web development.
TLDR: Install Google Chrome, Visual Studio Code, and npm
Here are links to the three tools that I think are vital to web development.
What tools are needed for web development?
A programmer of any sort needs two basic tools:
- A tool to write code.
- A to to run the code they have written.
Code is usually represented as text, so to write code you will need a text editor. Today most programmers use a text editor that has been adapted for the task of writing code called an Integrated Development Environment, or IDE. The IDE that most programmers prefer in 2023 is Visual Studio Code. It's developed by Microsoft, who has a long history of creating high quality products for developers.
Traditionally, code is compiled and run by a computer's operating system, however, many modern systems take code written by a developer, then run it live using a tool called an interpreter. In this series, we're going to be writing JavaScript code, then running it in the interpreter in Google Chrome or in Node.
So those are the three tools we need to install to setup a basic environment for writing code for the web:
Node also includes a tool that is fundamental to web development called Node Package Manager, or NPM for short. NPM is a tool for downloading chunks of code written by other programmers, or packages, and using them in your own app.
More
Here are some other resources and perspectives on setting up your computer for web programming.
Introduction to HTML
In this post, I'm going to introduce HTML, and demonstrate how it acts as the skeleton for all pages on the internet.
TLDR: HTML defines the structure of the page
HTML is used to define the structure of a web page. It's the structure that your styles (css), and code (JavaScript) sits on top of. The CSS styles the html elements. The code allows interaction with html elements.
What is HTML?
HTML stands for Hyper Text Markup Language. The unusual phrase "hyper text" means "more than text". Hyper text can be contrasted to plain text. You read plain text in a printed book. The words string together to form a story. But what if you want to know more about a topic? What if you want a definition for a term? What if you want to email the author? HTML gives web programmers the ability to "mark up" the text in a way that allows web browsers to add additional functionality to a text document.
HTML is one of the key inventions that enabled the web to become so popular. The ability to add cross-references directly in a document enables all sorts of connections and interactions that were formerly impossible. Nearly every page on the internet is made using HTML.
What are HTML elements?
The classic example of a hyper text element is a link. When links were invented, they were called "anchors", as if the author is anchoring a bit of text to another document. When you want to add a link to an html document you use the anchor or "a" element. Here's an example.
<a href='https://www.myblog.com'>My blog</a>
Wow, that's pretty confusing, isn't it? But take a moment to look more closely. What parts of it do you see?
An html element can have an opening tag, any number of attributes, content, and a closing tag, as shown in this diagram.
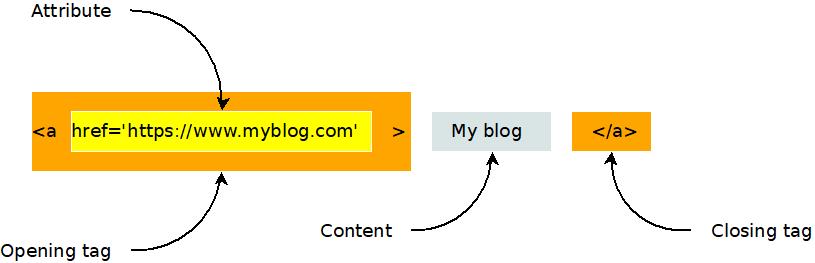
Here's another example of an html element. It's the image, or "i" element.
<img src="https://www.evanxmerz.com/images/AnchorTag_01.jpg" alt="Anchor tag diagram"/>
Notice that the image element shown here doesn't have a closing tag. This is the shortened form of an html element that doesn't need any content. It ends with "/>" and omits the content and closing tag.
The structure of an html document
HTML documents are composed of numerous html elements in a text file. The HTML elements are used to lay out the page in a way that the web browser can understand.
<!DOCTYPE html>
This must be the first line in every modern html document. It tells web browsers that the document is an html document.
<HTML>
The html element tells the browser where the HTML begins and ends.
<HEAD>
The head element tells the browser about what other resources are needed by this document. This includes styles, javascript files, fonts, and more.
<BODY>
The body element contains the content that will actually be shown to a user. This is where you put the stuff that you want to show to the user.
An example html document
Here's an example html document that contains all of the basic elements that are required to lay out a simple HTML document.
I want you to do something strange with this example. I want you to duplicate it by creating an html document on your computer and typing in the text in this example. Don't copy-paste it. When you copy-paste text, you are only learning how to copy-paste. That won't help you learn to be a programmer. Type this example into a new file. This helps you remember the code and gets you accustomed to actually being a programmer.
What about typos though? Wouldn't it be easier and faster to copy-paste? Yes, it would be easier and faster to copy-paste, however, typos are a natural part of the programming experience. Learning the patience required to hunt down typos is part of the journey.
Here are step-by-step instructions for how to do this exercise on a Windows computer.
- Open Windows Explorer
- Navigate to a folder for your html exercises
- Right click within the folder.
- Hover on "New"
- Click "Text Document"
- Rename the file to "hello_world.html"
- Right click on the new file.
- Hover over "Open With"
- Click Visual Studio Code. You must have installed Visual Studio Code to do this.
Now type the following text into the document.
<!DOCTYPE html>
<html>
<head>
<title>Hello, World in HTML</title>
</head>
<body>
Hello, World!
</body>
</html>
Congratulations, you just wrote your first web page!
Save it and double click it in Windows Explorer. It should open in Google Chrome, and look something like this.
There are many HTML elements
There are all sorts of different html elements. There's the "p" element for holding a paragraph of text, the "br" element for making line breaks, and the "style" element for incorporating inline css styles. Later in this text we will study some additional elements. For making basic web pages, we're going to focus on two elements: "div" and "span".
If you want to dig deeper into some other useful HTML elements, see my post on other useful html elements.
What is a div? What is a span?
That first example is pretty artificial. The body of the page only contains the text "Hello, World!". In a typical web page, there would be additional html elements in the body that structure the content of the page. This is where the div and span elements come in handy!
In the "div" element, the "div" stands for Content Division. Each div element should contain something on the page that should be separated from other things on the page. So if you had a menu on the page, you might put that in a div, then you might put the blog post content into a separate div.
The key thing to remember about the "div" element is that it is displayed in block style by default. This means that each div element creates a separate block of content from the others, typically by using a line break.
<div>This is some page content.</div>
<div>This is a menu or something.</div>
The "span" element differs from the div element in that it doesn't create a new section. The span element can be used to wrap elements that should be next to each other.
<span>This element</span> should be on the same line as <span>this element</span>.
An example will make this more clear.
Another example html document
Again, I want you to type this into a new html file on your computer. Call the file "divs_and_spans.html". Copy-pasting it won't help you learn.
<!DOCTYPE html>
<html>
<head>
<title>Divs and spans</title>
</head>
<body>
<div>
This is a div and <span>this is a span</span>.
</div>
<div>
This is another div and <span>this is another span</span>.
</div>
</body>
</html>
Here's what your page should look like.
It's not very exciting, is it? But most modern webpages are built using primarily the elements I've introduced to you on this page. The power of html isn't so much in the expressivity of the elements, but in how they can be combined in unique, interesting, and hierarchical ways to create new structures.
Summary
In this section I introduced Hyper Text Markup Language, aka HTML. Here are the key points to remember.
- HTML is the skeleton of a web page.
- HTML elements usually have opening and closing tags, as well as attributes.
- HTML documents use the head and body elements to structure the page.
- Div and span elements can be used to structure the body of your webpage.
More
Introduction to CSS
In this post, I'm going to introduce CSS, and demonstrate how it can be used to apply styles to a web page.
TLDR: CSS defines the look and feel of a web page
After building the structure of a page using HTML, you can add styles to HTML elements using CSS. If HTML is a car's frame, then CSS is the body shape, the paint job, and the rims.
What is CSS?
CSS stands for Cascading Style Sheets. Programmers and web designers use CSS to describe the colors, fonts, and positions of HTML elements. Web browsers interpret the CSS on a page to show those styles to viewers.
CSS can be added to an HTML document in multiple ways. It can be added directly to HTML elements using inline styles, it can be added to the head section using style elements, or it can be included from another file.
A simple inline css example
Here's an example of a very simple use of inline css using the style attribute on an element. All it does is set the font color to blue.
<div style="color: blue;">This text should be blue.</div>
To try this in your own html file, just type that code within the body section of the page. If you did it right, then the text should appear blue.
There are three important parts of this inline css. The first is the style attribute on the div element. Use the style attribute to apply inline styles. The second is the word "color", which specifies which property of the element that should be styled. The third important bit is the word "blue", which specifies the color. There are many ways to specify colors in CSS, and using names is the simplest.
I'm introducing inline styles here because they are useful for debugging, however, inline styles are impractical for a number of reasons, so we're going to look at a few other ways of incorporating CSS into an HTML document.
A simple CSS style element example
CSS styles can also be included in a document using the HTML style element. Usually such styles are placed in the head section of the document.
Using the HTML style element like this is generally discouraged, however, because the styles are not reusable in other documents. That's why styles are usually stored in separate files that can be included in any HTML document.
It's good to know about style elements, however, because they are sometimes used in emails. When creating a beautiful email, you don't have access to other files, so style elements are sometimes the best option.
<style>
span {
background-color: red;
}
</style>
This CSS rule sets the background color to red for all span elements on a page. Here's how it might look in a full HTML document.
<!DOCTYPE html>
<html>
<head>
<title>Divs and spans</title>
<style>
span {
background-color: red;
}
</style>
</head>
<body>
<div>
This is a div and <span>this is a span</span>.
</div>
<div>
Notice that <span>all span elements on the page receive the style indicated in the rule above</span>. This is due to how CSS selectors work.
</div>
</body>
</html>
Here's a graphic showing how that style breaks down into parts. The selector is used to select which elements will recieve the style. The property and value describe the style to apply, just like when using inline styles.
What are selectors?
The problem with inline CSS is that it clutters up the code for your webpage, and it's not reusable. A single style declaration can be twenty or more lines long. That's a lot to insert into an HTML element, and adding it makes the code difficult to read. Also, inline styles only apply to the element they are on. If you want to use an inline style twice, then you have to duplicate it, which is a big no-no in programming. Fortunately, there are other ways to write CSS.
CSS can be included in a "style" HTML element. CSS written in the style element uses syntax called "selectors" to apply styles to specific elements on a page.
For example, say you wanted all span elements on your page to have a larger font. You might include something like the following in the head section of your HTML document.
<style>
span {
font-size: 2em;
}
</style>
That selector selects all elements with a given tag name. You could use "div" to style all divs, or "h1" to style all h1 elements. But what if you want to be more specific what if you only want to style some elements of a given type?
If you want to be more specific, then you can add class or id attributes to your html, then use those attributes in your CSS selectors.
Here's an example that shows a class attribute on a div and a selector that uses the class value. Notice that the class name is preceded by a period in the selector.
<style>
div.blueText {
color: blue;
}
</style>
<div class="blueText">This text should be blue</div>
Here's an example that shows how to use the id attribute and selector. Notice that the hashtag symbol precedes the id in the css selector
<style>
#blueText {
color: blue;
}
</style>
<div id="blueText">This text should be blue</div>
Usually class is used when you want to have many things that share the same style. The id attribute is used when you are styling something that is unique on the page.
Including CSS in a separate file
Inline styles and style elements are not the most common way of including styles in a web page. Usually styles are imported from a stylesheet file that contains CSS and ends with the .css extension.
Type the following code into a file named css_example.css.
span.blue {
color: blue;
}
span.red {
color: red;
}
#title {
font-size: 2rem;
}
Now type the following code into a file named css_example.html.
<!DOCTYPE html>
<html>
<head>
<title>CSS Example</title>
<link rel="stylesheet" href="css_example.css">
</head>
<body>
<h1 id="title">This is a large title</h1>
<div>
This is a div with <span class="red">red text</span>.
</div>
<div>
This is another div with <span class="blue">blue text</span>.
</div>
</body>
</html>
If you did it all correctly, then you should end up with something like this, when opened in a web browser.
What are all the CSS properties?
CSS can be used to style just about any aspect of an HTML element. With CSS you can create shadows, animations, and nearly anything else you see on the web. In my posts I'm going to focus on the most common CSS properties, but if you want to see the complete list of things that can be styled with CSS, then check out MDN's comprehensive list of CSS properties.
Summary
- CSS is the style layer of the web.
- CSS is usually imported into an HTML file from a separate CSS file.
- The element, class, and id selectors allow you to target your styles to specific elements.
More
CSS is quite large and complex, but you don't have to know everything about it to use it effectively. You only need to know the fundamentals, and be able to use web search to find the other stuff.
Project: Personal web page
This post contains the first assignment in my free web programming course.
Project: Personal web page
Now that you know a little html and css, you should be able to produce some simple web pages. In this assignment, I want you to create a web page about yourself. It should be pretty similar in content to my about-me page on my website, but it should be about you and the things you like.
Here's what it must contain.
- A title that says your name and is styled like a title.
- A picture of you or something you like.
- A short paragraph of at least three sentences. You can use Lorem Ipsum if you can't write three sentences about yourself.
- A quote you like that is styled like a quotation. Usually quotes are indented more and displayed in italics.
- At least three links to things you've done or things you like. Use html lists to show these.
Other useful HTML elements
In this post, I'm going to introduce some HTML elements that are still useful in the modern web development, even if they aren't used as often as div or span.
TLDR: These HTML elements are useful for accessibility and search engine optimization
You can build most webpages using only div and span elements, along with the structural elements html, head, and body. However, it's important to know about other elements if you want your page to rank highly in Google search results, or you want your page to be accessible to people with disabilities.
Headings and titles
Heading elements are used to show titles or subtitles. They are important to your visitors because they make text more readable. They are important to Google because Google uses them to understand the content of your pages.
Heading elements are h1, h2, h3, h4, and h5. The h1 element is the top level title of the page, and should only occur once on a page, otherwise Google Search Console will show a warning for that page, and you won't rank as highly in web searches.
Here's an example of an h1 element.
<h1>I'm a page title that can be seen by the visitor</h1>
It's important to note that there IS an actual title element in HTML. When the title element appears in the header, it is used to set the text that is shown in the tab of your web browser.
<title>This is the text at the top of the tab</title>
Paragraphs in HTML
Before CSS was invented and standardized, there were lots of HTML elements used to format text. You can still use some of these, but it is widely discouraged. The "b" element bolds the enclosed text. The "i" element applies italics to the enclosed text. The "br" element adds a newline. You should never use any of those elements, but there is one element that is still quite useful.
The "p" tag is used to enclose a paragraph of text. It is useful as something separate from a div because it includes useful browser default styles, and because it serves as a good way to style the body text for your page.
<p>I'm a paragraph with some nice browser default styles.</p>
Browser defaults are a complex and controversial topic. Each browser starts with its own set of default styles so that a basic HTML page will be readable. There are many modern packages that override or eliminate these styles so that they don't interfere with a site's custom styles. I don't have a strong opinion on browser defaults in either direction.
Lists in HTML
Lists are another bit of semantic HTML that are still widely used. There are two types of lists in HTML, ordered lists and unordered lists. These can be represented by the "ol" and "ul" elements respectively. List items can be represented by the "li" element.
So what's the difference between the two list types? Ordered lists are numbered, while unordered lists are bulleted.
<ol>
<li>First item</li>
<li>Second item</li>
</ol>
If you drop that into an HTML file then you will see something like the following.
I encourage you to replace the "ol" elements with "ul" and see what happens.
Nav, main, and footer elements in HTML
Finally, I want to recommend using the elements nav, main, and footer. These elements are used to encapsulate the navigation portion of the page, the main content of the page, and the footer of the page. These elements are absolutely VITAL for accessibility, as they tell screen readers about which part of the page they are reading. You need to remember accessibility for two reasons. It is important to allow as many people as possible to access your site, and Google will penalize you if you don't.
They are used just like divs.
<nav>
<ul>
<li>Menu item 1</li>
<li>Menu item 2</li>
<li>etc</li>
</ul>
</nav>
Introduction to JavaScript
In this post, I'm going to introduce the JavaScript programming language and show you a few ways it's commonly used on the web.
WARNING: Some prior programming required!
I'm not introducing programming in general. This isn't a good first tutorial for someone who has never written a line of code in their life. I'm assuming that you know what functions are, and that methods and subroutines are the same thing. I'm assuming that you've written at least a little of some kind of code in the past.
TLDR: JavaScript is the engine of a webpage
HTML is used to define the structure of a web page. CSS defines the look and feel of a page. JavaScript defines how a page acts and responds to user input.
If a web page is a car, then HTML is the frame, CSS is the body, and JavaScript is the engine.
A haiku about JavaScript
Dearest Javascript,
You are my favorite tool
that costs me nothing.
Why not Java?
JavaScript has nothing to do with Java. The name JavaScript came about as an attempt to steal some of the hype around the Java programming language in the 1990s. In the 30 years since then, their positions have entirely flipped, with JavaScript now being the programming language that sees tons of innovation and market support, while Java is withering. Why has this happened? Why has JavaScript been so successful?
In my opinion, Java has been uniquely unsuccessful as a language because it has refused to grow and innovate. Java set some standards and some conventions for the language, and even thirty years later, the committees who guide specification for that language continue to be far too conservative.
If you're a Java programmer and you don't agree with me, then I encourage you to try Microsoft C#. That's the fun language that Java could have been.
And why is that?
C# and JavaScript have become incredibly open-ended. You can write code in lots of different ways, using features borrowed from other cool languages and frameworks. Both are sort of like Frankenstein's monster inasmuch as they are collections of mismatched parts that come together to form a surprisingly intimidating beast.
And this scares some people.
But, much like Shelley's actual monster, they are actually quite nice, and I hope to convey that to you in this introduction.
But what is JavaScript?
It's a programming language that runs in the web browser and on nearly every device that exists. On a webpage, you include JavaScript within the script element, or by including code in an external file. There's more to it than that, but let's learn by doing.
A JavaScript function
One of the key responsibilities of JavaScript is to respond to user input. Beside a few basic interactions, like clicking a link or a dropdown, every action that occurs on a webpage is handled by custom event handlers that are usually written in JavaScript. In this case, we're going to write a script that handles a click event.
When the user clicks on something, the browser creates what is called a click event. It then runs a bit of code called a function that is tied to that event. Here's the click handler function that we're going to write.
function showWarningOnClick() {
alert("Warning! The elders of the internet have been notified!");
}
That might look like nonsense to you, so let's break it down. This graphic shows how each of the pieces come together.
The first line is the function declaration. The word "function" declares that the next word is the name of a function. So "showWarningOnClick" is the name of the function. The parentheses, "()", would enclose the method arguments, if we needed any. The open bracket, "{", indicates that the next line will be the first line of the function body.
The second line is the actual code that is executed by this function. In this case, it calls another function. It calls "alert", with the argument "Warning! The elders of the internet have been notified!". Notice that the argument is enclosed with parentheses. Then the line ends with a semicolon. Most lines of JavaScript code must end in a semicolon.
That's the code that we will run when a button is clicked. We will see in the next section how we can trigger that function using a button.
A JavaScript event
The following line of code creates a button and adds a handler for the onClick event.
<button onClick="showWarningOnClick();">Don't click me</button>
Notice that the button isn't created using a div or span element, as you might expect. When creating buttons, it's good to use the button element if you can. This element is friendly to screen readers, and other accessibility technology.
The only attribute given is "onClick", and it's set to call the function that we defined earlier. The onClick attribute tells the browser what to do when the button is clicked.
It's important to note that onClick events can be attached to any elements. They aren't something unique to buttons. You can attach methods to the onClick attributes of divs or spans, or any other HTML elements.
Responding to a click event in JavaScript
So let's put it all together. The code should look something like this. And as a reminder, please type this in, don't copy paste it. The point is to learn, and you will learn and remember more if you type it out.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Hello World</title>
<script>
function showWarningOnClick() {
alert("Warning! The elders of the internet have been notified!");
}
</script>
</head>
<body>
<div>
<button onClick="showWarningOnClick();">Don't click me</button>
</div>
</body>
</html>
When you run it, by double clicking it and opening it in a web browser, you should see a single button in the upper left that says "DOn't click me". Click it, and you should see a little popup with the message "Warning! The elders of the internet have been notified!".
Summary
JavaScipt is a fun, open-ended programming language that is particularly useful in web browsers. In this post we learned about JavaScript functions and event handlers.
More
Here are some other introductions to JavaScript from around the web. I suggest that you spend time to check them out, even if you found this really easy. It's good to go over the fundamentals often, and it's particularly good to get multiple perspectives.
- MDN introduction to JavaScript. I link to MDN in every post because it's the most reliable source.
- Introduction to JavaScript from javascript.info.
- Introduction to JavaScript from freecodecamp. Some of what he says in this video is a bit misleading, but it's still a good place to start.
What is the DOM?
In this post, I'm going to introduce the Document Object Model, which is better known as the DOM.
TLDR: The DOM is the way a webpage is represented within a browser
The DOM is a bit of structured data that holds a representation of a webpage in order to enable programs to be able to manipulate it.
It's a bit like a 3d model that you might use in a 3d printer. The 3d printer is the display device, like a web browser. The 3d model is needed by the 3d printer for it to be able to do anything. You can manipulate the 3d model to make the 3d printer do what you want.
Why should we care about the DOM?
Why does the DOM matter? Can't we go about writing web code without knowing about the DOM?
The DOM matters because it is the representation of a web page that we can work with in JavaScript. It's true that, as a web programmer of over twenty years now, I have probably explicitly thought about the DOM only a handful of times. But I use it every day, and in the dark ages of JavaScript, we had to work with it very directly. These days we use packages like React or jQuery to wrap add a convenient layer of abstraction above the DOM. Still, in this post I'm going to show you how to use the DOM the old fashioned way.
This is still very important to know because occasionally you do have to do it, even when using the modern libraries for interacting with the DOM.
Finding elements in the DOM in JavaScript
In JavaScript, the DOM is stored as a global variable named "document". So when you want to do something with the DOM, you use the document variable.
One of the things you must be able to do is find the HTML element that you want to work with. The document gives us several handy methods to find elements by their id or class attributes. The getElementById method returns the first element found with the matching id attribute. Since id attributes should be unique, this should be the only element with that id in the document.
So to find a document with the id "elephant", you'd use the following line of code.
let elephantElement = document.getElementById("elephant");
This line of code contains a few things that might look unfamiliar to anyone who hasn't used JavaScript before. Here's a diagram that breaks it down.
The word "let" is a reserved word that indicates that the next word is a locally scoped variable. Don't worry if you don't know what "locally scoped" means. In this case, it just means that it's not a global variable, like document. Then the word "elephantElement" is the variable name, and the equals sign indicates that whatever is returned by the expression on the right should be stored in the variable on the left.
The id attribute isn't the only way to find elements. JavaScript also specifies a way to get elements with the same class attribute using the getElementsByClassName method. Notice that "Elements" is plural because the method returns an array, as many elements may share a class.
let elephantElements = document.getElementsByClassName("elephant");
The structure of this line of code is essentially the same as the previous one. The only difference is the name of the method that is called, the name of the variable, and the type of the variable.
How to modify a webpage using JavaScript
You can also use JavaScript to change the content of a webpage. When you've located the element you want to change, you can use the innerHTML method to get or set the content of that element. Like much of the JavaScript in this post, this is considered a bad thing to do these days. Typically you'd use the methods in a UI framework like React to mutate the page.
let elephantElement = document.getElementById("elephant");
elephantElement.innerHTML = "Is this an elephant?"
You can also add elements to the page using the createElement and append methods. This blurb appends a copyright notice to a blog post.
let blogPost = document.getElementById("post");
let copyrightNotice = document.createElement("p")
copyrightNotice.innerHTML = "Copyright " + new Date().getFullYear() + " by the author";
blogPost.append(copyrightNotice);
Let's put that into a real HTML file to see it in action. Here's the full example. If you've typed it in correctly, then you should see a copyright notice at the bottom of a paragraph of latin.
<!DOCTYPE html>
<html>
<head>
<title>How to modify the DOM using JavaScript</title>
</head>
<body>
<div id="post">
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
</div>
<script>
let blogPost = document.getElementById("post");
let copyrightNotice = document.createElement("p")
copyrightNotice.innerHTML = "Copyright " + new Date().getFullYear() + " by the author";
blogPost.append(copyrightNotice);
</script>
</body>
</html>
Summary
The DOM is the internal model of a webpage stored in the web browser. It can be manipulated using JavaScript methods such as getElementById, innerHTML, and append.
More
How to Debug a webpage in Chrome
In this post, I'm going to show you several ways to debug javascript in Google Chrome.
TLDR: Shift + ctrl + c
Open the javascript console using the shortcut shift + ctrl + c. That means, press all three at the same time. If you're on a Mac, use cmd rather than ctrl.
Inspecting an element to add new styles
Open the dom example that we wrote in What is the DOM?. In this post, we'll use that page to explore three ways to find out what's going on in a webpage. The code should look something like this.
<!DOCTYPE html>
<html>
<head>
<title>How to modify the DOM using JavaScript</title>
</head>
<body>
<div id="post">
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
</div>
<script>
let blogPost = document.getElementById("post");
let copyrightNotice = document.createElement("p")
copyrightNotice.innerHTML = "Copyright " + new Date().getFullYear() + " by the author";
blogPost.append(copyrightNotice);
</script>
</body>
</html>
After opening it in Chrome, right click on the copyright notice, then click "Inspect" in the popup menu. The html for the element you clicked should appear in the "Elements" tab of the browser console. It should look something like this but there are several places where the console can appear.
Any time you are confused about an element, inspecting it is a good place to start. In the browser console, you should be able to see the html for the element, as well as any attached css. In fact, you can actually add new styles using the browser console. Notice in the "Styles" tab where it says "element.style" and there are empty braces. Any CSS you type in there will be applied to the selected element. Styles can also be toggled on and off. This can be exceptionally handy when trying to figure out why your page doesn't look quite right.
Go ahead and try it. Enter "font-weight: 800" into element.style. What happens?
Debugging using console.log
The most basic way to debug JavaScript is to put some console.log statements into your code, then open the console and see what they print. Calling console.log tells the browser to print whatever you pass in in the JavaScript console. You can console.log just about anything, but the web browser will format variables automatically if you put them in an object using curly braces.
So the following console.log call isn't very useful.
console.log('HERE!');
Whereas the following console.log call will allow you to examine the object passed int.
console.log({ confusingVariable });
Add the following line of code to the dom example after it sets the copyrightNotice variable.
console.log({ copyrightNotice });
Then reload the page and use shift + ctrl + c to open the browser console, or shift + cmd + c on a Mac. You may have to expand the window and click over to the tab that says "Console".
You should see something that says "{ copyrightNotice: p }", and if you expand it, then you should be able to see all the properties associated with that variable. It's not particularly useful in this simple example, but I use this technique virtually every day as a web programmer.
Debugging using the Chrome debugger
Keep the dom example open in Chrome. Then press shift + ctrl + c to open the browser console. Navigate over to the "Sources" tab. This tab is the built in debugger provided by Google Chrome. In this debugger, you can add breakpoints to your code, and the browser will stop at the breakpoints and allow you to examine the state of your code. It's okay if this looks very confusing. We don't need to do much with it.
There are three things you need to be able to do. First, you need to be able to navigate to the sources tab. Then you need to find the line numbers, because you need to click them. This is much more difficult in a typical web page that has many files. Finally, you need to know how to continue running the page after it stops at your breakpoints.
After you've opened up the page, click line 12 to set a breakpoint there. In this case, that code has already been run, so reload the page to make it run again. When you do so, you should see something like the following.
Notice the area that I've circled in that screenshot. It's where you can see the state of your code. All of your variables should appear there and you should also see their values. Notice that copyrightNotice is undefined in this case. That's because Chrome pauses before running the code at the line where you placed your breakpoint. If you place a breakpoint on the next line, then press the play/pause button to resume execution, the value for copyrightNotice should be filled in.
The Chrome debugger is a great way to examine the state of your code.
Project: Client-side web form
This post contains the second assignment in my free web programming course.
Project: Client-side web form
Forms are how users enter data on the web. When you register for a website, you enter your username and email into a web form. When you buy an item from a web store, you send your payment information using a web form.
Generally speaking web forms have input components on them like text boxes and dropdowns and buttons. But this isn't always the case. Web forms can be cleverly hidden using JavaScript and CSS.
Typically a web form submits data to a server, which processes that data and responds to the user with something interesting. For instance, you might submit your username and password and get back an authentication token that allows you to use the private portion of a website.
Requirements
I want you to build a single web page using html and javascript only.
It should have three things on it.
- A page title in h1 tags.
- A label that reads "title:".
- An input text box.
- A submit button.
When the button is clicked, the title of the page should change to the title entered by the user without reloading the page.
Also, the user input must validated. The user should be prevented from using dirty words like "MacOS" and "iphone". Don't let them use either of those words in any capitalization. If those words occur in the input, then a red error message should be displayed underneath the text box that reads "Please refrain from using dirty words such as 'MacOS' and 'iphone'.".
Extra credit
For extra credit, make the form beautiful using CSS. Be creative, but try to make something that a typical web user might like.
Goodbye Gatsby! Hello Eleventy!
For the last few years, I had been running this blog using Gatsby. In general, I liked Gatsby. I liked that it was so simple up front, but had such deep complexity under the hood. I liked that it supported React out of the box. I liked that it could generate websites in so many different ways.
I liked it enough that I made my own minimalist blog starter called Empress, which was a fully featured blog based on the tutorial but with several key features added. I used that for this blog for several years and I liked it.
But man, the leadership of Gatsby was bad. They took it in such weird directions. They tried and failed at so many weird features that the documentation, and the product itself just became a dumping ground.
It really went off the rails when they created Gatsby "themes" that had nothing to do with the colloquial use of "themes". Themes were really more like plugins, but they were already using that term, too. So it was a hot mess, and soon enough the feature seemed to disappear from the docs and from existence.
Then they were bought by Next. I still don't understand that acquisition. Next.js is a good product with sensible leadership. I liked using it at my prior job, and I considered using it for this site, but it was just overkill.
So when I could no longer navigate the crazy web of dependencies to upgrade my blog to the latest version of Gatsby, I essentially stopped writing. I was just so frustrated with it.
Until this weekend, when I decided it was time to change.
Hello, Eleventy!
I decided to switch to Eleventy mostly because it's the static site generator with the most hype right now. Sometimes it's good to try the tech that is currently being hyped. It's good to stay up to date and have that line on your resume.
But also, Eleventy is a good product. I planned on spending a few weekends getting my blog switched over, but it only took me a few hours on Saturday, Sunday, and Monday to get it 95% switched over. There are still a few minor bugs here and there - don't check the title tags on tag pages, and ignore the reverse chronological sorting in some places - but in general the switch was very easy.
I've been around web programming since the 1990s, and Eleventy feels like a return to an older way of working, but with some of the good infrastructural tech we picked up along the way. Like now I'm writing in markdown, and I don't need to run MySQL on my server, nor do I even need to run a traditional "server". I can just host my static site on S3, and use GitHub Actions to deploy it, just like with Gatsby.
And I've heard some of the blowback against Jamstack architecture sites in the last few years. It's why I briefly considered using Next for this. But I still think JavaScript + API is the best/fastest/cleanest way to run a website, whether it's a blog or something more complex. I don't have an API for this site, but I feel like I could attach one without too much trouble if the need arose.
I do wish that I could use React. I saw some people try to get Eleventy working with React experimentally, but I didn't see any updated package to do it. Nunjuks and markdown are okay for the basics, but I do think that a true component-based architecture is ultimately a cleaner way to build UIs than a template based architecture.
Until next time...
So I'm going to give Eleventy a shot for now.
But we all know that those of us who still quixotically maintain personal blogs in 2024 are on a perpetual upgrade cycle. We all know that no "upgrade" is the final upgrade.
I wonder what I'll "upgrade" to in a few years? Maybe Wordpress will come back around?